数据分析与可视化 实践基础练习二(NumPy)
数据分析与可视化 实践基础练习二(NumPy)
一、Numpy相关函数或属性
- 数组的索引和切片
- 数组的运算
1.Numpy的一元函数
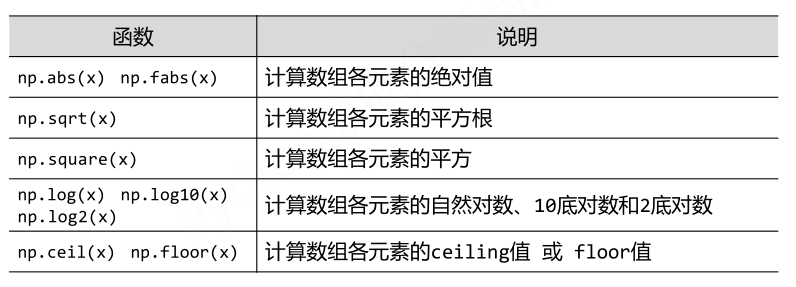

2.Numpy的二元函数

- 数组的读/写
1.np.savetxt() 和np.loadtxt()
np.savetxt() np.loadtxt()只能有效存取一维和二维数组
(1). np.savetxt()


(2). np.loadtxt()
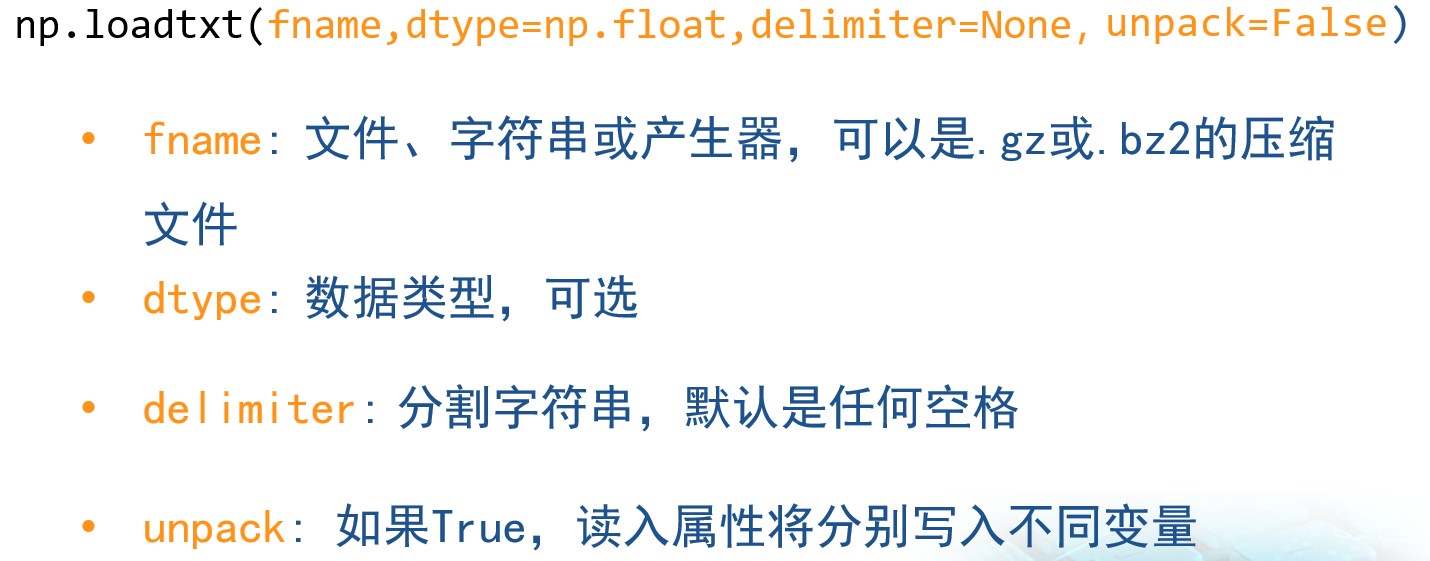
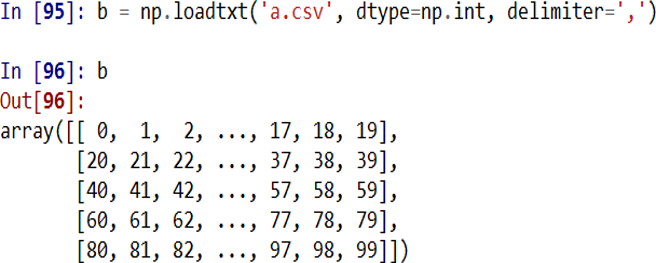
2.tofile() 和 np.fromfile()
任意维度的存取, 该方法需要读取时知道存入文件时数组的维度和元素类型
(1). tofile()
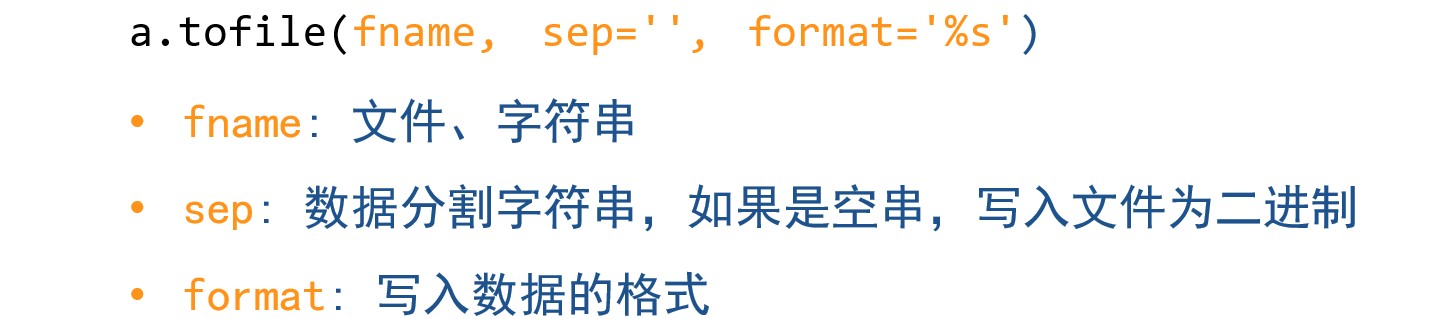

(2). np.fromfile()

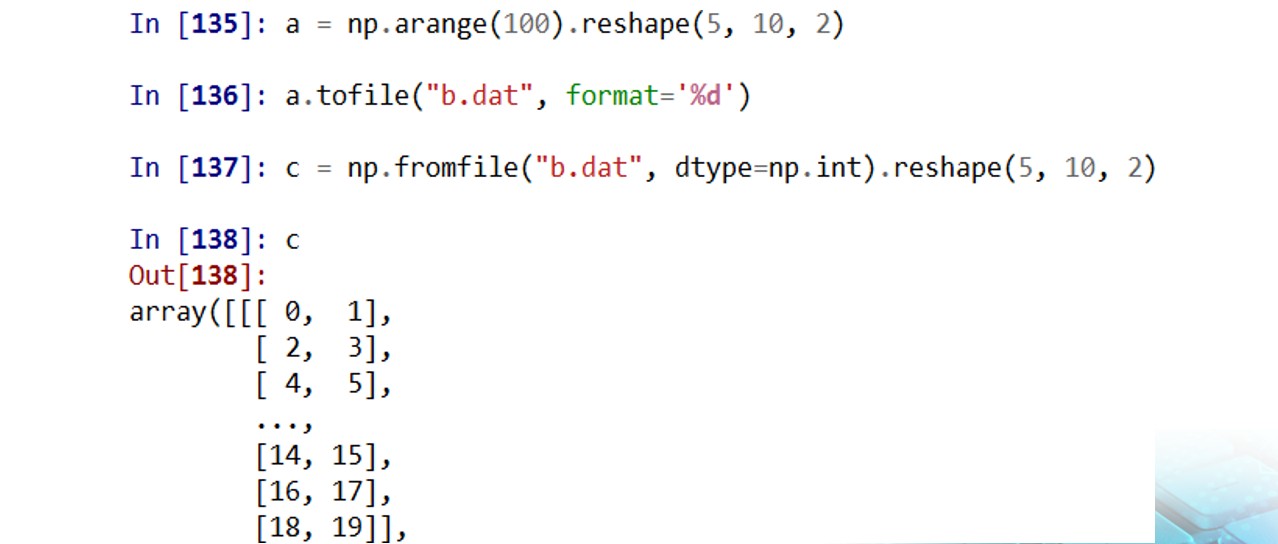
3.np.save()、np.savez() 和np.load()
numpy的便捷文件存取
(1). np.save() 和 np.load()


(2). np.savez()
1 | np.savez(file, *args, **kwds) |
以未压缩的.npz
格式将多个数组保存到一个文件中。
提供数组作为关键字参数,以将它们存储在输出文件中的相应名称下:savez(fn, x=x, y=y)
。
二、练习题
从数组np.arange(15)中提取5到10之间的所有数字 .
交换数组np.arange(9).reshape(3,3)中的第1列和第2列.
交换数组np.arange(9).reshape(3,3)中的第1行和第2行.
获取数组a = np.array([1,2,3,2,3,4,3,4,5,6])和数组b = np.array([7,2,10,2,7,4,9,4,9,8])之间的共同元素.
查找数组np.array([1,2,3,2,3,4,3,4,5,6])中的唯一值的数量.
查找二维数组np.arange(9).reshape(3,3)每一行中的最大值.
计算数组a = np.array([1,2,3,2,3,4,3,4,5,6])和数组b = np.array([7,2,10,2,7,4,9,4,9,8])之间的欧式距离.
查找数组np.array([7,2,10,2,7,4,9,4,9,8])中的第二大值.
三、题解
1 | # 1.从数组np.arange(15)中提取5到10之间的所有数字 |
a: [ 5 6 7 8 9 10]
1 | # 2.交换数组np.arange(9).reshape(3,3)中的第1列和第2列 |
[[1 0 2]
[4 3 5]
[7 6 8]]
1 | # 3.交换数组np.arange(9).reshape(3,3)中的第1行和第2行 |
[[3 4 5]
[0 1 2]
[6 7 8]]
1 | # 4.获取数组a = np.array([1,2,3,2,3,4,3,4,5,6])和数组b = np.array([7,2,10,2,7,4,9,4,9,8])之间的共同元素 |
[2 4]
1 | # 5.查找数组np.array([1,2,3,2,3,4,3,4,5,6])中的唯一值的数量 |
[1 2 3 4 5 6]
[1 2 3 2 1 1]
1 | # 6.查找二维数组np.arange(9).reshape(3,3)每一行中的最大值 |
[[2]
[5]
[8]]
[2 5 8]
1 | # 7.计算数组a = np.array([1,2,3,2,3,4,3,4,5,6])和数组b = np.array([7,2,10,2,7,4,9,4,9,8])之间的欧式距离 |
12.529964086141668
12.529964086141668
1 | # 8.查找数组np.array([7,2,10,2,7,4,9,4,9,8])中的第二大值 |
[ 2 2 4 4 7 7 8 9 9 10]
9