计算机视觉 上机实践一 图像的基本操作
计算机视觉 上机实践一 图像的基本操作
一、实验目的
通过本次实验,掌握图像的读取、显示、保存、绘制等基本操作;
熟悉图像的灰度直方图原理,并对图像进行灰度变换和颜色空间变换;
能绘制自定义图像。
二、实验环境
硬件环境:一台笔电
软件环境:Windows10环境、Jupyter Notebook软件;
三、实验内容及代码实现
1. 读取、显示、保存图像
源文件lena.png:

1 | # 1.读取、显示、保存Lena图像;(使用matplotlib库) |
2.直方图均衡化
内容:选择一张有明暗对比的图片(网上找一张即可),读取并绘制图像的直方图;对输入图像进行直方图均衡化并输出结果;
(1)使用Matplotlib库实现
1 | # 2.选择一张有明暗对比的图片,读取并绘制图像的直方图;对输入图像进行直方图均衡化并输出结果;(使用Matplotlib库实现) |
(2)使用opencv库实现
1 | # 2.更好的阅读体验,使用subplot创建单个子图(使用opencv库实现) |

3. 对图像进行灰度变换、颜色空间转换等基本操作
1 | # 3.对读入图像进行灰度变换、颜色空间转换等基本操作; |
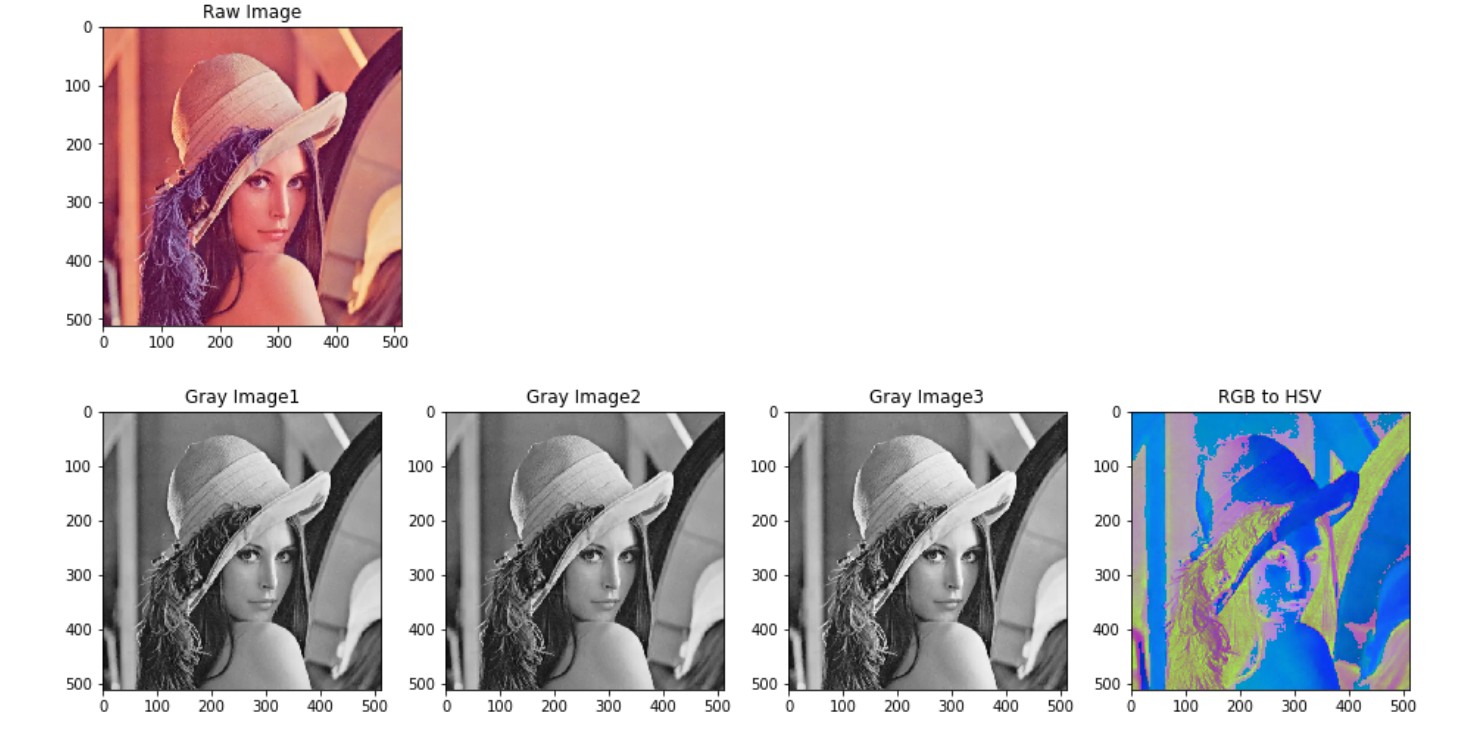
4. 自定义图像绘制
内容:定义一个像素为512*512的图像平面,黑色背景,在该图像上面生成一个正方形和圆,要求正方形的四个点为A(256,64)、B(256,256)、C(448,256)、D(448,64);要求圆的半径r=100,圆心为(256,256)。
1 | # 4.自定义图像绘制。定义一个像素为512*512的图像平面,黑色背景,在该图像上面生成一个正方形和圆, |
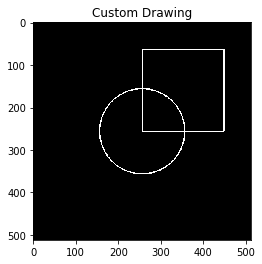
四、参考资料
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 AriesfunのBlog!
评论